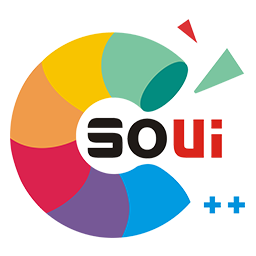 |
soui 5.0.0.1
Soui5 Doc
|
|
Loading...
Searching...
No Matches
Go to the documentation of this file.
18#define SOUI_ATTRS_BEGIN() \
20 virtual HRESULT SetAttribute(const SNS::SStringW &strAttribName, const SNS::SStringW &strValue, BOOL bLoading = FALSE) \
22 HRESULT hRet = E_FAIL;
25#define SOUI_ATTRS_END() \
27 return __baseCls::SetAttribute(strAttribName, strValue, bLoading); \
28 return AfterAttribute(strAttribName.c_str(), strValue.c_str(), bLoading, hRet); \
32#define SOUI_ATTRS_BREAK() \
37#define ATTR_CHAIN(varname, flag) \
38 if (FAILED(hRet) && SUCCEEDED(hRet = varname.SetAttribute(strAttribName, strValue, bLoading))) \
44#define ATTR_CHAIN_PTR(varname, flag) \
45 if (FAILED(hRet) && varname != NULL && SUCCEEDED(hRet = varname->ISetAttribute(&strAttribName, &strValue, bLoading))) \
51#define ATTR_CHAIN_CLASS(cls) \
53 hRet = cls::SetAttribute(strAttribName, strValue, bLoading);
55#define ATTR_CUSTOM(attribname, func) \
56 if (0 == strAttribName.CompareNoCase(attribname)) \
58 hRet = func(strValue, bLoading); \
62#define STRINGASBOOL(strValue) (strValue).CompareNoCase(L"0") != 0 && (strValue).CompareNoCase(L"false") != 0
64#define ATTR_BOOL(attribname, varname, allredraw) \
65 if (0 == strAttribName.CompareNoCase(attribname)) \
67 varname = STRINGASBOOL(strValue); \
68 hRet = allredraw ? S_OK : S_FALSE; \
73#define ATTR_INT(attribname, varname, allredraw) \
74 if (0 == strAttribName.CompareNoCase(attribname)) \
76 int nRet = Str2IntW(strValue, TRUE); \
78 hRet = allredraw ? S_OK : S_FALSE; \
82#define ATTR_LAYOUTSIZE(attribname, varname, allredraw) \
83 if (0 == strAttribName.CompareNoCase(attribname)) \
85 varname = GETLAYOUTSIZE(strValue); \
86 hRet = allredraw ? S_OK : S_FALSE; \
90#define ATTR_LAYOUTSIZE2(attribname, varname, allredraw) \
91 if (0 == strAttribName.CompareNoCase(attribname)) \
93 SStringWList values; \
94 if (SplitString(strValue, L',', values) != 2) \
95 return E_INVALIDARG; \
96 varname[0] = GETLAYOUTSIZE(values[0]); \
97 varname[1] = GETLAYOUTSIZE(values[1]); \
98 hRet = allredraw ? S_OK : S_FALSE; \
102#define ATTR_LAYOUTSIZE4(attribname, varname, allredraw) \
103 if (0 == strAttribName.CompareNoCase(attribname)) \
105 SStringWList values; \
106 if (SplitString(strValue, L',', values) != 4) \
107 return E_INVALIDARG; \
108 varname[0] = GETLAYOUTSIZE(values[0]); \
109 varname[1] = GETLAYOUTSIZE(values[1]); \
110 varname[2] = GETLAYOUTSIZE(values[2]); \
111 varname[3] = GETLAYOUTSIZE(values[3]); \
112 hRet = allredraw ? S_OK : S_FALSE; \
116#define ATTR_MARGIN(attribname, varname, allredraw) \
117 if (0 == strAttribName.CompareNoCase(attribname)) \
119 SStringWList values; \
120 if (SplitString(strValue, L',', values) != 4) \
121 return E_INVALIDARG; \
122 varname.left = GETLAYOUTSIZE(values[0]).toPixelSize(100); \
123 varname.top = GETLAYOUTSIZE(values[1]).toPixelSize(100); \
124 varname.right = GETLAYOUTSIZE(values[2]).toPixelSize(100); \
125 varname.bottom = GETLAYOUTSIZE(values[3]).toPixelSize(100); \
126 hRet = allredraw ? S_OK : S_FALSE; \
131#define ATTR_RECT(attribname, varname, allredraw) \
132 if (0 == strAttribName.CompareNoCase(attribname)) \
134 swscanf_s(strValue, L"%d,%d,%d,%d", &varname.left, &varname.top, &varname.right, &varname.bottom); \
135 hRet = allredraw ? S_OK : S_FALSE; \
140#define ATTR_SIZE(attribname, varname, allredraw) \
141 if (0 == strAttribName.CompareNoCase(attribname)) \
143 swscanf_s(strValue, L"%d,%d", &varname.cx, &varname.cy); \
144 hRet = allredraw ? S_OK : S_FALSE; \
149#define ATTR_POINT(attribname, varname, allredraw) \
150 if (0 == strAttribName.CompareNoCase(attribname)) \
152 swscanf_s(strValue, L"%d,%d", &varname.x, &varname.y); \
153 hRet = allredraw ? S_OK : S_FALSE; \
158#define ATTR_SPOINT(attribname, varname, allredraw) \
159 if (0 == strAttribName.CompareNoCase(attribname)) \
161 swscanf_s(strValue, L"%f,%f", &varname.fX, &varname.fY); \
162 hRet = allredraw ? S_OK : S_FALSE; \
167#define ATTR_FLOAT(attribname, varname, allredraw) \
168 if (0 == strAttribName.CompareNoCase(attribname)) \
170 swscanf_s(strValue, L"%f", &varname); \
171 hRet = allredraw ? S_OK : S_FALSE; \
176#define ATTR_UINT(attribname, varname, allredraw) \
177 if (0 == strAttribName.CompareNoCase(attribname)) \
179 int nRet = Str2IntW(strValue, TRUE); \
180 varname = (UINT)nRet; \
181 hRet = allredraw ? S_OK : S_FALSE; \
186#define ATTR_DWORD(attribname, varname, allredraw) \
187 if (0 == strAttribName.CompareNoCase(attribname)) \
189 int nRet = Str2IntW(strValue, TRUE); \
190 varname = (DWORD)nRet; \
191 hRet = allredraw ? S_OK : S_FALSE; \
196#define ATTR_WORD(attribname, varname, allredraw) \
197 if (0 == strAttribName.CompareNoCase(attribname)) \
199 int nRet = Str2IntW(strValue, TRUE); \
200 varname = (WORD)nRet; \
201 hRet = allredraw ? S_OK : S_FALSE; \
206#define ATTR_BIT(attribname, varname, maskbit, allredraw) \
207 if (0 == strAttribName.CompareNoCase(attribname)) \
209 bool bSet = STRINGASBOOL(strValue); \
211 varname |= maskbit; \
213 varname &= ~(maskbit); \
214 hRet = allredraw ? S_OK : S_FALSE; \
219#define ATTR_STRINGA(attribname, varname, allredraw) \
220 if (0 == strAttribName.CompareNoCase(attribname)) \
222 SNS::SStringW strTmp = GETSTRING(strValue); \
223 varname = S_CW2A(strTmp); \
224 hRet = allredraw ? S_OK : S_FALSE; \
229#define ATTR_STRINGW(attribname, varname, allredraw) \
230 if (0 == strAttribName.CompareNoCase(attribname)) \
232 varname = GETSTRING(strValue); \
233 hRet = allredraw ? S_OK : S_FALSE; \
238#define ATTR_STRINGT(attribname, varname, allredraw) \
239 if (0 == strAttribName.CompareNoCase(attribname)) \
241 varname = S_CW2T(GETSTRING(strValue)); \
242 hRet = allredraw ? S_OK : S_FALSE; \
247#define ATTR_I18NSTRA(attribname, varname, allredraw) \
248 if (0 == strAttribName.CompareNoCase(attribname)) \
250 SNS::SStringW strTmp = tr(GETSTRING(strValue)); \
251 varname = S_CW2A(strTmp); \
252 hRet = allredraw ? S_OK : S_FALSE; \
257#define ATTR_I18NSTRT(attribname, varname, allredraw) \
258 if (0 == strAttribName.CompareNoCase(attribname)) \
260 SNS::SStringW strTmp = GETSTRING(strValue); \
261 varname.SetText(S_CW2T(strTmp)); \
262 hRet = allredraw ? S_OK : S_FALSE; \
267#define ATTR_HEX(attribname, varname, allredraw) \
268 if (0 == strAttribName.CompareNoCase(attribname)) \
270 int nRet = Str2IntW(strValue, TRUE); \
272 hRet = allredraw ? S_OK : S_FALSE; \
277#define ATTR_COLOR(attribname, varname, allredraw) \
278 if (0 == strAttribName.CompareNoCase(attribname)) \
280 if (!strValue.IsEmpty()) \
282 varname = GETCOLOR(strValue); \
283 hRet = allredraw ? S_OK : S_FALSE; \
293#define ATTR_FONT(attribname, varname, allredraw) \
294 if (0 == strAttribName.CompareNoCase(attribname)) \
296 varname.SetFontDesc(strValue, GetScale()); \
297 hRet = allredraw ? S_OK : S_FALSE; \
302#define ATTR_ENUM_BEGIN(attribname, vartype, allredraw) \
303 if (0 == strAttribName.CompareNoCase(attribname)) \
307 hRet = allredraw ? S_OK : S_FALSE;
309#define ATTR_ENUM_VALUE(enumstring, enumvalue) \
310 if (strValue == enumstring) \
311 varTemp = enumvalue; \
314#define ATTR_ENUM_END(varname) \
322#define ATTR_STYLE(attribname, varname, allredraw) \
323 if (0 == strAttribName.CompareNoCase(attribname)) \
325 GETSTYLE(strValue, varname); \
326 hRet = allredraw ? S_OK : S_FALSE; \
331#define ATTR_SKIN(attribname, varname, allredraw) \
332 if (0 == strAttribName.CompareNoCase(attribname)) \
334 varname = GETSKIN(strValue, GetScale()); \
335 hRet = allredraw ? S_OK : S_FALSE; \
340#define ATTR_INTERPOLATOR(attribname, varname, allredraw) \
341 if (0 == strAttribName.CompareNoCase(attribname)) \
343 varname.Attach(CREATEINTERPOLATOR(strValue)); \
344 hRet = allredraw ? S_OK : S_FALSE; \
350#define ATTR_IMAGE(attribname, varname, allredraw) \
351 if (0 == strAttribName.CompareNoCase(attribname)) \
353 SNS::IBitmapS *pImg = LOADIMAGE2(strValue); \
359 varname->Release(); \
361 hRet = allredraw ? S_OK : S_FALSE; \
367#define ATTR_IMAGEAUTOREF(attribname, varname, allredraw) \
368 if (0 == strAttribName.CompareNoCase(attribname)) \
370 SNS::IBitmapS *pImg = LOADIMAGE2(strValue); \
377 hRet = allredraw ? S_OK : S_FALSE; \
382#define ATTR_ICON(attribname, varname, allredraw) \
383 if (0 == strAttribName.CompareNoCase(attribname)) \
386 DestroyIcon(varname); \
387 varname = LOADICON2(strValue); \
388 hRet = allredraw ? S_OK : S_FALSE; \
392#define ATTR_CHAR(attribname, varname, allredraw) \
393 if (0 == strAttribName.CompareNoCase(attribname)) \
395 varname = *(LPCWSTR)strValue; \
396 hRet = allredraw ? S_OK : S_FALSE; \
400#define ATTR_ANIMATION(attribname, varname, allredraw) \
401 if (0 == strAttribName.CompareNoCase(attribname)) \
403 varname.Attach(SApplication::getSingleton().LoadAnimation(S_CW2T(strValue))); \
404 hRet = allredraw ? S_OK : S_FALSE; \
408#define ATTR_GRADIENT(attribname, varname, allredraw) \
409 if (0 == strAttribName.CompareNoCase(attribname)) \
411 SNS::IGradient *pGradient = GETUIDEF->GetGradient(strValue); \
416 varname = pGradient; \
417 hRet = allredraw ? S_OK : S_FALSE; \