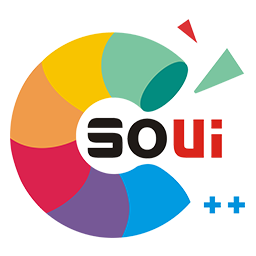 |
soui 5.0.0.1
Soui5 Doc
|
|
Loading...
Searching...
No Matches
12#ifndef __SWNDMSGCRACK_H__
13#define __SWNDMSGCRACK_H__
25#define DECLEAR_MSG_MAP_EX() \
29 BOOL IsMsgHandled() const \
31 return m_bMsgHandled; \
33 void SetMsgHandled(BOOL bHandled) \
35 m_bMsgHandled = bHandled; \
37 BOOL ProcessWindowMessage(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam, LRESULT& lResult, DWORD dwMsgMapID = 0) \
39 BOOL bOldMsgHandled = m_bMsgHandled; \
40 BOOL bRet = _ProcessWindowMessage(hWnd, uMsg, wParam, lParam, lResult, dwMsgMapID); \
41 m_bMsgHandled = bOldMsgHandled; \
44 BOOL _ProcessWindowMessage(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam, LRESULT& lResult, DWORD dwMsgMapID);
47#define BEGIN_MSG_MAP_EX2(theClass) \
48 BOOL theClass::_ProcessWindowMessage(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam, LRESULT& lResult, DWORD dwMsgMapID) \
50 BOOL bHandled = TRUE; \
61#define BEGIN_MSG_MAP_EX(theClass) \
65 BOOL IsMsgHandled() const \
67 return m_bMsgHandled; \
69 void SetMsgHandled(BOOL bHandled) \
71 m_bMsgHandled = bHandled; \
73 BOOL ProcessWindowMessage(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam, LRESULT& lResult, DWORD dwMsgMapID = 0) \
75 BOOL bOldMsgHandled = m_bMsgHandled; \
76 BOOL bRet = _ProcessWindowMessage(hWnd, uMsg, wParam, lParam, lResult, dwMsgMapID); \
77 m_bMsgHandled = bOldMsgHandled; \
80 BOOL _ProcessWindowMessage(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam, LRESULT& lResult, DWORD dwMsgMapID) \
82 BOOL bHandled = TRUE; \
95#define END_MSG_MAP() \
108#define REFLECT_NOTIFICATIONS_EX() \
111 lResult = ReflectNotifications(uMsg, wParam, lParam, bHandled); \
119#define REFLECT_NOTIFY_CODE(cd) \
120 if(uMsg == WM_NOTIFY && cd == ((LPNMHDR)lParam)->code) \
123 lResult = ReflectNotifications(uMsg, wParam, lParam, bHandled); \
129#define CHAIN_MSG_MAP(theChainClass) \
131 if(theChainClass::ProcessWindowMessage(hWnd, uMsg, wParam, lParam, lResult)) \
135#define CHAIN_MSG_MAP_MEMBER(theChainMember) \
137 if((theChainMember).ProcessWindowMessage(hWnd, uMsg, wParam, lParam, lResult)) \
141#define MESSAGE_HANDLER(msg, func) \
145 lResult = func(uMsg, wParam, lParam, bHandled); \
150#define ALT_MSG_MAP(msgMapID) \
158#define MSG_WM_CREATE(func) \
159 if (uMsg == WM_CREATE) \
161 SetMsgHandled(TRUE); \
162 lResult = (LRESULT)func((LPCREATESTRUCT)lParam); \
168#define MSG_WM_INITDIALOG(func) \
169 if (uMsg == WM_INITDIALOG) \
171 SetMsgHandled(TRUE); \
172 lResult = (LRESULT)func((HWND)wParam, lParam); \
178#define MSG_WM_COPYDATA(func) \
179 if (uMsg == WM_COPYDATA) \
181 SetMsgHandled(TRUE); \
182 lResult = (LRESULT)func((HWND)wParam, (PCOPYDATASTRUCT)lParam); \
188#define WM_DPICHANGED 0x02E0
191#define MSG_WM_DPICHANGED(func)\
192 if (uMsg == WM_DPICHANGED) \
194 SetMsgHandled(TRUE); \
195 func((WORD)HIWORD(wParam), (RECT* const)lParam); \
196 if (IsMsgHandled()) \
202#define MSG_WM_DESTROY(func) \
203 if (uMsg == WM_DESTROY) \
205 SetMsgHandled(TRUE); \
213#define MSG_WM_MOVE(func) \
214 if (uMsg == WM_MOVE) \
216 SetMsgHandled(TRUE); \
217 func(_WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
224#define MSG_WM_SIZE(func) \
225 if (uMsg == WM_SIZE) \
227 SetMsgHandled(TRUE); \
228 func((UINT)wParam, _WTYPES_NS::CSize(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
235#define MSG_WM_ACTIVATE(func) \
236 if (uMsg == WM_ACTIVATE) \
238 SetMsgHandled(TRUE); \
239 func((UINT)LOWORD(wParam), (BOOL)HIWORD(wParam), (HWND)lParam); \
246#define MSG_WM_SETFOCUS(func) \
247 if (uMsg == WM_SETFOCUS) \
249 SetMsgHandled(TRUE); \
250 func((HWND)wParam); \
257#define MSG_WM_KILLFOCUS(func) \
258 if (uMsg == WM_KILLFOCUS) \
260 SetMsgHandled(TRUE); \
261 func((HWND)wParam); \
268#define MSG_WM_ENABLE_EX(func) \
269 if (uMsg == WM_ENABLE) \
271 SetMsgHandled(TRUE); \
272 func((BOOL)wParam,(UINT)lParam); \
279#define MSG_WM_PAINT(func) \
280 if (uMsg == WM_PAINT) \
282 SetMsgHandled(TRUE); \
290#define MSG_WM_CLOSE(func) \
291 if (uMsg == WM_CLOSE) \
293 SetMsgHandled(TRUE); \
301#define MSG_WM_QUERYENDSESSION(func) \
302 if (uMsg == WM_QUERYENDSESSION) \
304 SetMsgHandled(TRUE); \
305 lResult = (LRESULT)func((UINT)wParam, (UINT)lParam); \
311#define MSG_WM_QUERYOPEN(func) \
312 if (uMsg == WM_QUERYOPEN) \
314 SetMsgHandled(TRUE); \
315 lResult = (LRESULT)func(); \
321#define MSG_WM_ERASEBKGND(func) \
322 if (uMsg == WM_ERASEBKGND) \
324 SetMsgHandled(TRUE); \
325 lResult = (LRESULT)func((HDC)wParam); \
331#define MSG_WM_SYSCOLORCHANGE(func) \
332 if (uMsg == WM_SYSCOLORCHANGE) \
334 SetMsgHandled(TRUE); \
342#define MSG_WM_ENDSESSION(func) \
343 if (uMsg == WM_ENDSESSION) \
345 SetMsgHandled(TRUE); \
346 func((BOOL)wParam, (UINT)lParam); \
353#define MSG_WM_SHOWWINDOW(func) \
354 if (uMsg == WM_SHOWWINDOW) \
356 SetMsgHandled(TRUE); \
357 func((BOOL)wParam, (int)lParam); \
364#define MSG_WM_CTLCOLOREDIT(func) \
365 if (uMsg == WM_CTLCOLOREDIT) \
367 SetMsgHandled(TRUE); \
368 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
374#define MSG_WM_CTLCOLORLISTBOX(func) \
375 if (uMsg == WM_CTLCOLORLISTBOX) \
377 SetMsgHandled(TRUE); \
378 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
384#define MSG_WM_CTLCOLORBTN(func) \
385 if (uMsg == WM_CTLCOLORBTN) \
387 SetMsgHandled(TRUE); \
388 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
394#define MSG_WM_CTLCOLORDLG(func) \
395 if (uMsg == WM_CTLCOLORDLG) \
397 SetMsgHandled(TRUE); \
398 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
404#define MSG_WM_CTLCOLORSCROLLBAR(func) \
405 if (uMsg == WM_CTLCOLORSCROLLBAR) \
407 SetMsgHandled(TRUE); \
408 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
414#define MSG_WM_CTLCOLORSTATIC(func) \
415 if (uMsg == WM_CTLCOLORSTATIC) \
417 SetMsgHandled(TRUE); \
418 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
424#define MSG_WM_SETTINGCHANGE(func) \
425 if (uMsg == WM_SETTINGCHANGE) \
427 SetMsgHandled(TRUE); \
428 func((UINT)wParam, (LPCTSTR)lParam); \
435#define MSG_WM_DEVMODECHANGE(func) \
436 if (uMsg == WM_DEVMODECHANGE) \
438 SetMsgHandled(TRUE); \
439 func((LPCTSTR)lParam); \
446#define MSG_WM_ACTIVATEAPP(func) \
447 if (uMsg == WM_ACTIVATEAPP) \
449 SetMsgHandled(TRUE); \
450 func((BOOL)wParam, (DWORD)lParam); \
457#define MSG_WM_FONTCHANGE(func) \
458 if (uMsg == WM_FONTCHANGE) \
460 SetMsgHandled(TRUE); \
468#define MSG_WM_TIMECHANGE(func) \
469 if (uMsg == WM_TIMECHANGE) \
471 SetMsgHandled(TRUE); \
479#define MSG_WM_CANCELMODE(func) \
480 if (uMsg == WM_CANCELMODE) \
482 SetMsgHandled(TRUE); \
490#define MSG_WM_SETCURSOR(func) \
491 if (uMsg == WM_SETCURSOR) \
493 SetMsgHandled(TRUE); \
494 lResult = (LRESULT)func((HWND)wParam, (UINT)LOWORD(lParam), (UINT)HIWORD(lParam)); \
500#define MSG_WM_MOUSEACTIVATE(func) \
501 if (uMsg == WM_MOUSEACTIVATE) \
503 SetMsgHandled(TRUE); \
504 lResult = (LRESULT)func((HWND)wParam, (UINT)LOWORD(lParam), (UINT)HIWORD(lParam)); \
510#define MSG_WM_CHILDACTIVATE(func) \
511 if (uMsg == WM_CHILDACTIVATE) \
513 SetMsgHandled(TRUE); \
521#define MSG_WM_GETMINMAXINFO(func) \
522 if (uMsg == WM_GETMINMAXINFO) \
524 SetMsgHandled(TRUE); \
525 func((LPMINMAXINFO)lParam); \
532#define MSG_WM_ICONERASEBKGND(func) \
533 if (uMsg == WM_ICONERASEBKGND) \
535 SetMsgHandled(TRUE); \
543#define MSG_WM_SPOOLERSTATUS(func) \
544 if (uMsg == WM_SPOOLERSTATUS) \
546 SetMsgHandled(TRUE); \
547 func((UINT)wParam, (UINT)LOWORD(lParam)); \
554#define MSG_WM_DRAWITEM(func) \
555 if (uMsg == WM_DRAWITEM) \
557 SetMsgHandled(TRUE); \
558 func((UINT)wParam, (LPDRAWITEMSTRUCT)lParam); \
565#define MSG_WM_MEASUREITEM(func) \
566 if (uMsg == WM_MEASUREITEM) \
568 SetMsgHandled(TRUE); \
569 func((UINT)wParam, (LPMEASUREITEMSTRUCT)lParam); \
576#define MSG_WM_DELETEITEM(func) \
577 if (uMsg == WM_DELETEITEM) \
579 SetMsgHandled(TRUE); \
580 func((UINT)wParam, (LPDELETEITEMSTRUCT)lParam); \
587#define MSG_WM_CHARTOITEM(func) \
588 if (uMsg == WM_CHARTOITEM) \
590 SetMsgHandled(TRUE); \
591 lResult = (LRESULT)func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), (HWND)lParam); \
597#define MSG_WM_VKEYTOITEM(func) \
598 if (uMsg == WM_VKEYTOITEM) \
600 SetMsgHandled(TRUE); \
601 lResult = (LRESULT)func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), (HWND)lParam); \
607#define MSG_WM_QUERYDRAGICON(func) \
608 if (uMsg == WM_QUERYDRAGICON) \
610 SetMsgHandled(TRUE); \
611 lResult = (LRESULT)func(); \
617#define MSG_WM_COMPAREITEM(func) \
618 if (uMsg == WM_COMPAREITEM) \
620 SetMsgHandled(TRUE); \
621 lResult = (LRESULT)func((UINT)wParam, (LPCOMPAREITEMSTRUCT)lParam); \
627#define MSG_WM_COMPACTING(func) \
628 if (uMsg == WM_COMPACTING) \
630 SetMsgHandled(TRUE); \
631 func((UINT)wParam); \
638#define MSG_WM_NCCREATE(func) \
639 if (uMsg == WM_NCCREATE) \
641 SetMsgHandled(TRUE); \
642 lResult = (LRESULT)func((LPCREATESTRUCT)lParam); \
648#define MSG_WM_NCDESTROY(func) \
649 if (uMsg == WM_NCDESTROY) \
651 SetMsgHandled(TRUE); \
659#define MSG_WM_NCCALCSIZE(func) \
660 if (uMsg == WM_NCCALCSIZE) \
662 SetMsgHandled(TRUE); \
663 lResult = func((BOOL)wParam, lParam); \
669#define MSG_WM_NCHITTEST(func) \
670 if (uMsg == WM_NCHITTEST) \
672 SetMsgHandled(TRUE); \
673 lResult = (LRESULT)func(_WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
679#define MSG_WM_NCPAINT(func) \
680 if (uMsg == WM_NCPAINT) \
682 SetMsgHandled(TRUE); \
683 func((HRGN)wParam); \
690#define MSG_WM_NCACTIVATE(func) \
691 if (uMsg == WM_NCACTIVATE) \
693 SetMsgHandled(TRUE); \
694 lResult = (LRESULT)func((BOOL)wParam); \
700#define MSG_WM_GETDLGCODE(func) \
701 if (uMsg == WM_GETDLGCODE) \
703 SetMsgHandled(TRUE); \
704 lResult = (LRESULT)func((LPMSG)lParam); \
710#define MSG_WM_NCMOUSEMOVE(func) \
711 if (uMsg == WM_NCMOUSEMOVE) \
713 SetMsgHandled(TRUE); \
714 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
721#define MSG_WM_NCLBUTTONDOWN(func) \
722 if (uMsg == WM_NCLBUTTONDOWN) \
724 SetMsgHandled(TRUE); \
725 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
732#define MSG_WM_NCLBUTTONUP(func) \
733 if (uMsg == WM_NCLBUTTONUP) \
735 SetMsgHandled(TRUE); \
736 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
743#define MSG_WM_NCLBUTTONDBLCLK(func) \
744 if (uMsg == WM_NCLBUTTONDBLCLK) \
746 SetMsgHandled(TRUE); \
747 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
754#define MSG_WM_NCRBUTTONDOWN(func) \
755 if (uMsg == WM_NCRBUTTONDOWN) \
757 SetMsgHandled(TRUE); \
758 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
765#define MSG_WM_NCRBUTTONUP(func) \
766 if (uMsg == WM_NCRBUTTONUP) \
768 SetMsgHandled(TRUE); \
769 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
776#define MSG_WM_NCRBUTTONDBLCLK(func) \
777 if (uMsg == WM_NCRBUTTONDBLCLK) \
779 SetMsgHandled(TRUE); \
780 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
787#define MSG_WM_NCMBUTTONDOWN(func) \
788 if (uMsg == WM_NCMBUTTONDOWN) \
790 SetMsgHandled(TRUE); \
791 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
798#define MSG_WM_NCMBUTTONUP(func) \
799 if (uMsg == WM_NCMBUTTONUP) \
801 SetMsgHandled(TRUE); \
802 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
809#define MSG_WM_NCMBUTTONDBLCLK(func) \
810 if (uMsg == WM_NCMBUTTONDBLCLK) \
812 SetMsgHandled(TRUE); \
813 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
820#define MSG_WM_KEYDOWN(func) \
821 if (uMsg == WM_KEYDOWN) \
823 SetMsgHandled(TRUE); \
824 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
831#define MSG_WM_KEYUP(func) \
832 if (uMsg == WM_KEYUP) \
834 SetMsgHandled(TRUE); \
835 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
842#define MSG_WM_CHAR(func) \
843 if (uMsg == WM_CHAR) \
845 SetMsgHandled(TRUE); \
846 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
853#define MSG_WM_DEADCHAR(func) \
854 if (uMsg == WM_DEADCHAR) \
856 SetMsgHandled(TRUE); \
857 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
864#define MSG_WM_SYSKEYDOWN(func) \
865 if (uMsg == WM_SYSKEYDOWN) \
867 SetMsgHandled(TRUE); \
868 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
875#define MSG_WM_SYSKEYUP(func) \
876 if (uMsg == WM_SYSKEYUP) \
878 SetMsgHandled(TRUE); \
879 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
886#define MSG_WM_SYSCHAR(func) \
887 if (uMsg == WM_SYSCHAR) \
889 SetMsgHandled(TRUE); \
890 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
897#define MSG_WM_SYSDEADCHAR(func) \
898 if (uMsg == WM_SYSDEADCHAR) \
900 SetMsgHandled(TRUE); \
901 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
908#define MSG_WM_SYSCOMMAND(func) \
909 if (uMsg == WM_SYSCOMMAND) \
911 SetMsgHandled(TRUE); \
912 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
919#define MSG_WM_TCARD(func) \
920 if (uMsg == WM_TCARD) \
922 SetMsgHandled(TRUE); \
923 func((UINT)wParam, (DWORD)lParam); \
930#define MSG_WM_TIMER(func) \
931 if (uMsg == WM_TIMER) \
933 SetMsgHandled(TRUE); \
934 func((UINT_PTR)wParam); \
941#define MSG_WM_HSCROLL(func) \
942 if (uMsg == WM_HSCROLL) \
944 SetMsgHandled(TRUE); \
945 func((int)LOWORD(wParam), (short)HIWORD(wParam), (HWND)lParam); \
952#define MSG_WM_VSCROLL(func) \
953 if (uMsg == WM_VSCROLL) \
955 SetMsgHandled(TRUE); \
956 func((int)LOWORD(wParam), (short)HIWORD(wParam), (HWND)lParam); \
963#define MSG_WM_INITMENU(func) \
964 if (uMsg == WM_INITMENU) \
966 SetMsgHandled(TRUE); \
967 func((HMENU)wParam); \
974#define MSG_WM_INITMENUPOPUP(func) \
975 if (uMsg == WM_INITMENUPOPUP) \
977 SetMsgHandled(TRUE); \
978 func((HMENU)wParam, (UINT)LOWORD(lParam), (BOOL)HIWORD(lParam)); \
985#define MSG_WM_INITMENUPOPUP_EX(func) \
986 if (uMsg == WM_INITMENUPOPUP) \
988 SetMsgHandled(TRUE); \
989 func((SMenuEx*)wParam, (int)(lParam)); \
996#define MSG_WM_MENUSELECT(func) \
997 if (uMsg == WM_MENUSELECT) \
999 SetMsgHandled(TRUE); \
1000 func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), (HMENU)lParam); \
1002 if(IsMsgHandled()) \
1007#define MSG_WM_MENUCHAR(func) \
1008 if (uMsg == WM_MENUCHAR) \
1010 SetMsgHandled(TRUE); \
1011 lResult = func((TCHAR)LOWORD(wParam), (UINT)HIWORD(wParam), (HMENU)lParam); \
1012 if(IsMsgHandled()) \
1017#define MSG_WM_NOTIFY(func) \
1018 if (uMsg == WM_NOTIFY) \
1020 SetMsgHandled(TRUE); \
1021 lResult = func((int)wParam, (LPNMHDR)lParam); \
1022 if(IsMsgHandled()) \
1027#define MSG_WM_ENTERIDLE(func) \
1028 if (uMsg == WM_ENTERIDLE) \
1030 SetMsgHandled(TRUE); \
1031 func((UINT)wParam, (HWND)lParam); \
1033 if(IsMsgHandled()) \
1038#define MSG_WM_MOUSEMOVE(func) \
1039 if (uMsg == WM_MOUSEMOVE) \
1041 SetMsgHandled(TRUE); \
1042 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1044 if(IsMsgHandled()) \
1049#define MSG_WM_MOUSEWHEEL(func) \
1050 if (uMsg == WM_MOUSEWHEEL) \
1052 SetMsgHandled(TRUE); \
1053 lResult = (LRESULT)func((UINT)LOWORD(wParam), (short)HIWORD(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1054 if(IsMsgHandled()) \
1059#define MSG_WM_LBUTTONDOWN(func) \
1060 if (uMsg == WM_LBUTTONDOWN) \
1062 SetMsgHandled(TRUE); \
1063 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1065 if(IsMsgHandled()) \
1070#define MSG_WM_LBUTTONUP(func) \
1071 if (uMsg == WM_LBUTTONUP) \
1073 SetMsgHandled(TRUE); \
1074 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1076 if(IsMsgHandled()) \
1081#define MSG_WM_LBUTTONDBLCLK(func) \
1082 if (uMsg == WM_LBUTTONDBLCLK) \
1084 SetMsgHandled(TRUE); \
1085 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1087 if(IsMsgHandled()) \
1092#define MSG_WM_RBUTTONDOWN(func) \
1093 if (uMsg == WM_RBUTTONDOWN) \
1095 SetMsgHandled(TRUE); \
1096 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1098 if(IsMsgHandled()) \
1103#define MSG_WM_RBUTTONUP(func) \
1104 if (uMsg == WM_RBUTTONUP) \
1106 SetMsgHandled(TRUE); \
1107 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1109 if(IsMsgHandled()) \
1114#define MSG_WM_RBUTTONDBLCLK(func) \
1115 if (uMsg == WM_RBUTTONDBLCLK) \
1117 SetMsgHandled(TRUE); \
1118 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1120 if(IsMsgHandled()) \
1125#define MSG_WM_MBUTTONDOWN(func) \
1126 if (uMsg == WM_MBUTTONDOWN) \
1128 SetMsgHandled(TRUE); \
1129 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1131 if(IsMsgHandled()) \
1136#define MSG_WM_MBUTTONUP(func) \
1137 if (uMsg == WM_MBUTTONUP) \
1139 SetMsgHandled(TRUE); \
1140 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1142 if(IsMsgHandled()) \
1147#define MSG_WM_MBUTTONDBLCLK(func) \
1148 if (uMsg == WM_MBUTTONDBLCLK) \
1150 SetMsgHandled(TRUE); \
1151 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1153 if(IsMsgHandled()) \
1158#define MSG_WM_PARENTNOTIFY(func) \
1159 if (uMsg == WM_PARENTNOTIFY) \
1161 SetMsgHandled(TRUE); \
1162 func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), lParam); \
1164 if(IsMsgHandled()) \
1169#define MSG_WM_MDIACTIVATE(func) \
1170 if (uMsg == WM_MDIACTIVATE) \
1172 SetMsgHandled(TRUE); \
1173 func((HWND)wParam, (HWND)lParam); \
1175 if(IsMsgHandled()) \
1180#define MSG_WM_RENDERFORMAT(func) \
1181 if (uMsg == WM_RENDERFORMAT) \
1183 SetMsgHandled(TRUE); \
1184 func((UINT)wParam); \
1186 if(IsMsgHandled()) \
1191#define MSG_WM_RENDERALLFORMATS(func) \
1192 if (uMsg == WM_RENDERALLFORMATS) \
1194 SetMsgHandled(TRUE); \
1197 if(IsMsgHandled()) \
1202#define MSG_WM_DESTROYCLIPBOARD(func) \
1203 if (uMsg == WM_DESTROYCLIPBOARD) \
1205 SetMsgHandled(TRUE); \
1208 if(IsMsgHandled()) \
1213#define MSG_WM_DRAWCLIPBOARD(func) \
1214 if (uMsg == WM_DRAWCLIPBOARD) \
1216 SetMsgHandled(TRUE); \
1219 if(IsMsgHandled()) \
1224#define MSG_WM_PAINTCLIPBOARD(func) \
1225 if (uMsg == WM_PAINTCLIPBOARD) \
1227 SetMsgHandled(TRUE); \
1228 func((HWND)wParam, (const LPPAINTSTRUCT)::GlobalLock((HGLOBAL)lParam)); \
1229 ::GlobalUnlock((HGLOBAL)lParam); \
1231 if(IsMsgHandled()) \
1236#define MSG_WM_VSCROLLCLIPBOARD(func) \
1237 if (uMsg == WM_VSCROLLCLIPBOARD) \
1239 SetMsgHandled(TRUE); \
1240 func((HWND)wParam, (UINT)LOWORD(lParam), (UINT)HIWORD(lParam)); \
1242 if(IsMsgHandled()) \
1247#define MSG_WM_CONTEXTMENU(func) \
1248 if (uMsg == WM_CONTEXTMENU) \
1250 SetMsgHandled(TRUE); \
1251 func((HWND)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1253 if(IsMsgHandled()) \
1258#define MSG_WM_SIZECLIPBOARD(func) \
1259 if (uMsg == WM_SIZECLIPBOARD) \
1261 SetMsgHandled(TRUE); \
1262 func((HWND)wParam, (const LPRECT)::GlobalLock((HGLOBAL)lParam)); \
1263 ::GlobalUnlock((HGLOBAL)lParam); \
1265 if(IsMsgHandled()) \
1270#define MSG_WM_ASKCBFORMATNAME(func) \
1271 if (uMsg == WM_ASKCBFORMATNAME) \
1273 SetMsgHandled(TRUE); \
1274 func((DWORD)wParam, (LPTSTR)lParam); \
1276 if(IsMsgHandled()) \
1281#define MSG_WM_CHANGECBCHAIN(func) \
1282 if (uMsg == WM_CHANGECBCHAIN) \
1284 SetMsgHandled(TRUE); \
1285 func((HWND)wParam, (HWND)lParam); \
1287 if(IsMsgHandled()) \
1292#define MSG_WM_HSCROLLCLIPBOARD(func) \
1293 if (uMsg == WM_HSCROLLCLIPBOARD) \
1295 SetMsgHandled(TRUE); \
1296 func((HWND)wParam, (UINT)LOWORD(lParam), (UINT)HIWORD(lParam)); \
1298 if(IsMsgHandled()) \
1303#define MSG_WM_QUERYNEWPALETTE(func) \
1304 if (uMsg == WM_QUERYNEWPALETTE) \
1306 SetMsgHandled(TRUE); \
1307 lResult = (LRESULT)func(); \
1308 if(IsMsgHandled()) \
1313#define MSG_WM_PALETTECHANGED(func) \
1314 if (uMsg == WM_PALETTECHANGED) \
1316 SetMsgHandled(TRUE); \
1317 func((HWND)wParam); \
1319 if(IsMsgHandled()) \
1324#define MSG_WM_PALETTEISCHANGING(func) \
1325 if (uMsg == WM_PALETTEISCHANGING) \
1327 SetMsgHandled(TRUE); \
1328 func((HWND)wParam); \
1330 if(IsMsgHandled()) \
1335#define MSG_WM_DROPFILES(func) \
1336 if (uMsg == WM_DROPFILES) \
1338 SetMsgHandled(TRUE); \
1339 func((HDROP)wParam); \
1341 if(IsMsgHandled()) \
1346#define MSG_WM_WINDOWPOSCHANGING(func) \
1347 if (uMsg == WM_WINDOWPOSCHANGING) \
1349 SetMsgHandled(TRUE); \
1350 func((LPWINDOWPOS)lParam); \
1352 if(IsMsgHandled()) \
1357#define MSG_WM_WINDOWPOSCHANGED(func) \
1358 if (uMsg == WM_WINDOWPOSCHANGED) \
1360 SetMsgHandled(TRUE); \
1361 func((LPWINDOWPOS)lParam); \
1363 if(IsMsgHandled()) \
1368#define MSG_WM_EXITMENULOOP(func) \
1369 if (uMsg == WM_EXITMENULOOP) \
1371 SetMsgHandled(TRUE); \
1372 func((BOOL)wParam); \
1374 if(IsMsgHandled()) \
1379#define MSG_WM_ENTERMENULOOP(func) \
1380 if (uMsg == WM_ENTERMENULOOP) \
1382 SetMsgHandled(TRUE); \
1383 func((BOOL)wParam); \
1385 if(IsMsgHandled()) \
1390#define MSG_WM_STYLECHANGED(func) \
1391 if (uMsg == WM_STYLECHANGED) \
1393 SetMsgHandled(TRUE); \
1394 func((UINT)wParam, (LPSTYLESTRUCT)lParam); \
1396 if(IsMsgHandled()) \
1401#define MSG_WM_STYLECHANGING(func) \
1402 if (uMsg == WM_STYLECHANGING) \
1404 SetMsgHandled(TRUE); \
1405 func((UINT)wParam, (LPSTYLESTRUCT)lParam); \
1407 if(IsMsgHandled()) \
1412#define MSG_WM_SIZING(func) \
1413 if (uMsg == WM_SIZING) \
1415 SetMsgHandled(TRUE); \
1416 func((UINT)wParam, (LPRECT)lParam); \
1418 if(IsMsgHandled()) \
1423#define MSG_WM_MOVING(func) \
1424 if (uMsg == WM_MOVING) \
1426 SetMsgHandled(TRUE); \
1427 func((UINT)wParam, (LPRECT)lParam); \
1429 if(IsMsgHandled()) \
1434#define MSG_WM_CAPTURECHANGED(func) \
1435 if (uMsg == WM_CAPTURECHANGED) \
1437 SetMsgHandled(TRUE); \
1438 func((HWND)lParam); \
1440 if(IsMsgHandled()) \
1445#define MSG_WM_DEVICECHANGE(func) \
1446 if (uMsg == WM_DEVICECHANGE) \
1448 SetMsgHandled(TRUE); \
1449 lResult = (LRESULT)func((UINT)wParam, (DWORD)lParam); \
1450 if(IsMsgHandled()) \
1455#define MSG_WM_COMMAND(func) \
1456 if (uMsg == WM_COMMAND) \
1458 SetMsgHandled(TRUE); \
1459 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
1461 if(IsMsgHandled()) \
1466#define MSG_WM_DISPLAYCHANGE(func) \
1467 if (uMsg == WM_DISPLAYCHANGE) \
1469 SetMsgHandled(TRUE); \
1470 func((UINT)wParam, _WTYPES_NS::CSize(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1472 if(IsMsgHandled()) \
1477#define MSG_WM_ENTERSIZEMOVE(func) \
1478 if (uMsg == WM_ENTERSIZEMOVE) \
1480 SetMsgHandled(TRUE); \
1483 if(IsMsgHandled()) \
1488#define MSG_WM_EXITSIZEMOVE(func) \
1489 if (uMsg == WM_EXITSIZEMOVE) \
1491 SetMsgHandled(TRUE); \
1494 if(IsMsgHandled()) \
1499#define MSG_WM_GETFONT(func) \
1500 if (uMsg == WM_GETFONT) \
1502 SetMsgHandled(TRUE); \
1503 lResult = (LRESULT)func(); \
1504 if(IsMsgHandled()) \
1509#define MSG_WM_GETHOTKEY(func) \
1510 if (uMsg == WM_GETHOTKEY) \
1512 SetMsgHandled(TRUE); \
1514 if(IsMsgHandled()) \
1519#define MSG_WM_GETICON(func) \
1520 if (uMsg == WM_GETICON) \
1522 SetMsgHandled(TRUE); \
1523 lResult = (LRESULT)func((UINT)wParam); \
1524 if(IsMsgHandled()) \
1529#define MSG_WM_GETTEXT(func) \
1530 if (uMsg == WM_GETTEXT) \
1532 SetMsgHandled(TRUE); \
1533 lResult = (LRESULT)func((int)wParam, (LPTSTR)lParam); \
1534 if(IsMsgHandled()) \
1539#define MSG_WM_GETTEXTLENGTH(func) \
1540 if (uMsg == WM_GETTEXTLENGTH) \
1542 SetMsgHandled(TRUE); \
1543 lResult = (LRESULT)func(); \
1544 if(IsMsgHandled()) \
1549#define MSG_WM_HELP(func) \
1550 if (uMsg == WM_HELP) \
1552 SetMsgHandled(TRUE); \
1553 func((LPHELPINFO)lParam); \
1555 if(IsMsgHandled()) \
1560#define MSG_WM_HOTKEY(func) \
1561 if (uMsg == WM_HOTKEY) \
1563 SetMsgHandled(TRUE); \
1564 func((int)wParam, (UINT)LOWORD(lParam), (UINT)HIWORD(lParam)); \
1566 if(IsMsgHandled()) \
1571#define MSG_WM_INPUTLANGCHANGE(func) \
1572 if (uMsg == WM_INPUTLANGCHANGE) \
1574 SetMsgHandled(TRUE); \
1575 func((DWORD)wParam, (HKL)lParam); \
1577 if(IsMsgHandled()) \
1582#define MSG_WM_INPUTLANGCHANGEREQUEST(func) \
1583 if (uMsg == WM_INPUTLANGCHANGEREQUEST) \
1585 SetMsgHandled(TRUE); \
1586 func((BOOL)wParam, (HKL)lParam); \
1588 if(IsMsgHandled()) \
1593#define MSG_WM_NEXTDLGCTL(func) \
1594 if (uMsg == WM_NEXTDLGCTL) \
1596 SetMsgHandled(TRUE); \
1597 func((BOOL)LOWORD(lParam), wParam); \
1599 if(IsMsgHandled()) \
1604#define MSG_WM_NEXTMENU(func) \
1605 if (uMsg == WM_NEXTMENU) \
1607 SetMsgHandled(TRUE); \
1608 func((int)wParam, (LPMDINEXTMENU)lParam); \
1610 if(IsMsgHandled()) \
1615#define MSG_WM_NOTIFYFORMAT(func) \
1616 if (uMsg == WM_NOTIFYFORMAT) \
1618 SetMsgHandled(TRUE); \
1619 lResult = (LRESULT)func((HWND)wParam, (int)lParam); \
1620 if(IsMsgHandled()) \
1625#define MSG_WM_POWERBROADCAST(func) \
1626 if (uMsg == WM_POWERBROADCAST) \
1628 SetMsgHandled(TRUE); \
1629 lResult = (LRESULT)func((DWORD)wParam, (DWORD)lParam); \
1630 if(IsMsgHandled()) \
1635#define MSG_WM_PRINT(func) \
1636 if (uMsg == WM_PRINT) \
1638 SetMsgHandled(TRUE); \
1639 func((HDC)wParam, (UINT)lParam); \
1641 if(IsMsgHandled()) \
1646#define MSG_WM_PRINTCLIENT(func) \
1647 if (uMsg == WM_PRINTCLIENT) \
1649 SetMsgHandled(TRUE); \
1650 func((HDC)wParam, (UINT)lParam); \
1652 if(IsMsgHandled()) \
1657#define MSG_WM_RASDIALEVENT(func) \
1658 if (uMsg == WM_RASDIALEVENT) \
1660 SetMsgHandled(TRUE); \
1661 func((RASCONNSTATE)wParam, (DWORD)lParam); \
1663 if(IsMsgHandled()) \
1668#define MSG_WM_SETFONT(func) \
1669 if (uMsg == WM_SETFONT) \
1671 SetMsgHandled(TRUE); \
1672 func((HFONT)wParam, (BOOL)LOWORD(lParam)); \
1674 if(IsMsgHandled()) \
1679#define MSG_WM_SETHOTKEY(func) \
1680 if (uMsg == WM_SETHOTKEY) \
1682 SetMsgHandled(TRUE); \
1683 lResult = (LRESULT)func((int)LOBYTE(LOWORD(wParam)), (UINT)HIBYTE(LOWORD(wParam))); \
1684 if(IsMsgHandled()) \
1689#define MSG_WM_SETICON(func) \
1690 if (uMsg == WM_SETICON) \
1692 SetMsgHandled(TRUE); \
1693 lResult = (LRESULT)func((UINT)wParam, (HICON)lParam); \
1694 if(IsMsgHandled()) \
1699#define MSG_WM_SETREDRAW(func) \
1700 if (uMsg == WM_SETREDRAW) \
1702 SetMsgHandled(TRUE); \
1703 func((BOOL)wParam); \
1705 if(IsMsgHandled()) \
1710#define MSG_WM_SETTEXT(func) \
1711 if (uMsg == WM_SETTEXT) \
1713 SetMsgHandled(TRUE); \
1714 lResult = (LRESULT)func((LPCTSTR)lParam); \
1715 if(IsMsgHandled()) \
1720#define MSG_WM_USERCHANGED(func) \
1721 if (uMsg == WM_USERCHANGED) \
1723 SetMsgHandled(TRUE); \
1726 if(IsMsgHandled()) \
1735#define MSG_WM_MOUSEHOVER(func) \
1736 if (uMsg == WM_MOUSEHOVER) \
1738 SetMsgHandled(TRUE); \
1739 func((UINT)wParam, _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1741 if(IsMsgHandled()) \
1746#define MSG_WM_MOUSELEAVE(func) \
1747 if (uMsg == WM_MOUSELEAVE) \
1749 SetMsgHandled(TRUE); \
1752 if(IsMsgHandled()) \
1758#define MSG_WM_MENURBUTTONUP(func) \
1759 if (uMsg == WM_MENURBUTTONUP) \
1761 SetMsgHandled(TRUE); \
1762 func(wParam, (HMENU)lParam); \
1764 if(IsMsgHandled()) \
1769#define MSG_WM_MENUDRAG(func) \
1770 if (uMsg == WM_MENUDRAG) \
1772 SetMsgHandled(TRUE); \
1773 lResult = func(wParam, (HMENU)lParam); \
1774 if(IsMsgHandled()) \
1779#define MSG_WM_MENUGETOBJECT(func) \
1780 if (uMsg == WM_MENUGETOBJECT) \
1782 SetMsgHandled(TRUE); \
1783 lResult = func((PMENUGETOBJECTINFO)lParam); \
1784 if(IsMsgHandled()) \
1789#define MSG_WM_UNINITMENUPOPUP(func) \
1790 if (uMsg == WM_UNINITMENUPOPUP) \
1792 SetMsgHandled(TRUE); \
1793 func((UINT)HIWORD(lParam), (HMENU)wParam); \
1795 if(IsMsgHandled()) \
1800#define MSG_WM_MENUCOMMAND(func) \
1801 if (uMsg == WM_MENUCOMMAND) \
1803 SetMsgHandled(TRUE); \
1804 func(wParam, (HMENU)lParam); \
1806 if(IsMsgHandled()) \
1812#define MSG_WM_APPCOMMAND(func) \
1813 if (uMsg == WM_APPCOMMAND) \
1815 SetMsgHandled(TRUE); \
1816 lResult = (LRESULT)func((HWND)wParam, GET_APPCOMMAND_LPARAM(lParam), GET_DEVICE_LPARAM(lParam), GET_KEYSTATE_LPARAM(lParam)); \
1817 if(IsMsgHandled()) \
1822#define MSG_WM_NCXBUTTONDOWN(func) \
1823 if (uMsg == WM_NCXBUTTONDOWN) \
1825 SetMsgHandled(TRUE); \
1826 func(GET_XBUTTON_WPARAM(wParam), GET_NCHITTEST_WPARAM(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1828 if(IsMsgHandled()) \
1833#define MSG_WM_NCXBUTTONUP(func) \
1834 if (uMsg == WM_NCXBUTTONUP) \
1836 SetMsgHandled(TRUE); \
1837 func(GET_XBUTTON_WPARAM(wParam), GET_NCHITTEST_WPARAM(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1839 if(IsMsgHandled()) \
1844#define MSG_WM_NCXBUTTONDBLCLK(func) \
1845 if (uMsg == WM_NCXBUTTONDBLCLK) \
1847 SetMsgHandled(TRUE); \
1848 func(GET_XBUTTON_WPARAM(wParam), GET_NCHITTEST_WPARAM(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1850 if(IsMsgHandled()) \
1855#define MSG_WM_XBUTTONDOWN(func) \
1856 if (uMsg == WM_XBUTTONDOWN) \
1858 SetMsgHandled(TRUE); \
1859 func(GET_XBUTTON_WPARAM(wParam), GET_KEYSTATE_WPARAM(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1861 if(IsMsgHandled()) \
1866#define MSG_WM_XBUTTONUP(func) \
1867 if (uMsg == WM_XBUTTONUP) \
1869 SetMsgHandled(TRUE); \
1870 func(GET_XBUTTON_WPARAM(wParam), GET_KEYSTATE_WPARAM(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1872 if(IsMsgHandled()) \
1877#define MSG_WM_XBUTTONDBLCLK(func) \
1878 if (uMsg == WM_XBUTTONDBLCLK) \
1880 SetMsgHandled(TRUE); \
1881 func(GET_XBUTTON_WPARAM(wParam), GET_KEYSTATE_WPARAM(wParam), _WTYPES_NS::CPoint(GET_X_LPARAM(lParam), GET_Y_LPARAM(lParam))); \
1883 if(IsMsgHandled()) \
1888#define MSG_WM_CHANGEUISTATE(func) \
1889 if (uMsg == WM_CHANGEUISTATE) \
1891 SetMsgHandled(TRUE); \
1892 func(LOWORD(wParam), HIWORD(wParam)); \
1894 if(IsMsgHandled()) \
1899#define MSG_WM_UPDATEUISTATE(func) \
1900 if (uMsg == WM_UPDATEUISTATE) \
1902 SetMsgHandled(TRUE); \
1903 func(LOWORD(wParam), HIWORD(wParam)); \
1905 if(IsMsgHandled()) \
1910#define MSG_WM_QUERYUISTATE(func) \
1911 if (uMsg == WM_QUERYUISTATE) \
1913 SetMsgHandled(TRUE); \
1915 if(IsMsgHandled()) \
1920#define MSG_WM_INPUT(func) \
1921 if (uMsg == WM_INPUT) \
1923 SetMsgHandled(TRUE); \
1924 func(GET_RAWINPUT_CODE_WPARAM(wParam), (HRAWINPUT)lParam); \
1926 if(IsMsgHandled()) \
1931#define MSG_WM_UNICHAR(func) \
1932 if (uMsg == WM_UNICHAR) \
1934 SetMsgHandled(TRUE); \
1935 func((TCHAR)wParam, (UINT)lParam & 0xFFFF, (UINT)((lParam & 0xFFFF0000) >> 16)); \
1936 if(IsMsgHandled()) \
1938 lResult = (wParam == UNICODE_NOCHAR) ? TRUE : FALSE; \
1944#define MSG_WM_WTSSESSION_CHANGE(func) \
1945 if (uMsg == WM_WTSSESSION_CHANGE) \
1947 SetMsgHandled(TRUE); \
1948 func(wParam, (PWTSSESSION_NOTIFICATION)lParam); \
1950 if(IsMsgHandled()) \
1955#define MSG_WM_THEMECHANGED(func) \
1956 if (uMsg == WM_THEMECHANGED) \
1958 SetMsgHandled(TRUE); \
1961 if(IsMsgHandled()) \
1970#define MSG_WM_FORWARDMSG(func) \
1971 if (uMsg == WM_FORWARDMSG) \
1973 SetMsgHandled(TRUE); \
1974 lResult = (LRESULT)func((LPMSG)lParam, (DWORD)wParam); \
1975 if(IsMsgHandled()) \
1983#define MSG_DM_GETDEFID(func) \
1984 if (uMsg == DM_GETDEFID) \
1986 SetMsgHandled(TRUE); \
1988 if(IsMsgHandled()) \
1993#define MSG_DM_SETDEFID(func) \
1994 if (uMsg == DM_SETDEFID) \
1996 SetMsgHandled(TRUE); \
1997 func((UINT)wParam); \
1999 if(IsMsgHandled()) \
2004#define MSG_DM_REPOSITION(func) \
2005 if (uMsg == DM_REPOSITION) \
2007 SetMsgHandled(TRUE); \
2010 if(IsMsgHandled()) \
2018#define MSG_OCM_COMMAND(func) \
2019 if (uMsg == OCM_COMMAND) \
2021 SetMsgHandled(TRUE); \
2022 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2024 if(IsMsgHandled()) \
2029#define MSG_OCM_NOTIFY(func) \
2030 if (uMsg == OCM_NOTIFY) \
2032 SetMsgHandled(TRUE); \
2033 lResult = func((int)wParam, (LPNMHDR)lParam); \
2034 if(IsMsgHandled()) \
2039#define MSG_OCM_PARENTNOTIFY(func) \
2040 if (uMsg == OCM_PARENTNOTIFY) \
2042 SetMsgHandled(TRUE); \
2043 func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), lParam); \
2045 if(IsMsgHandled()) \
2050#define MSG_OCM_DRAWITEM(func) \
2051 if (uMsg == OCM_DRAWITEM) \
2053 SetMsgHandled(TRUE); \
2054 func((UINT)wParam, (LPDRAWITEMSTRUCT)lParam); \
2056 if(IsMsgHandled()) \
2061#define MSG_OCM_MEASUREITEM(func) \
2062 if (uMsg == OCM_MEASUREITEM) \
2064 SetMsgHandled(TRUE); \
2065 func((UINT)wParam, (LPMEASUREITEMSTRUCT)lParam); \
2067 if(IsMsgHandled()) \
2072#define MSG_OCM_COMPAREITEM(func) \
2073 if (uMsg == OCM_COMPAREITEM) \
2075 SetMsgHandled(TRUE); \
2076 lResult = (LRESULT)func((UINT)wParam, (LPCOMPAREITEMSTRUCT)lParam); \
2077 if(IsMsgHandled()) \
2082#define MSG_OCM_DELETEITEM(func) \
2083 if (uMsg == OCM_DELETEITEM) \
2085 SetMsgHandled(TRUE); \
2086 func((UINT)wParam, (LPDELETEITEMSTRUCT)lParam); \
2088 if(IsMsgHandled()) \
2093#define MSG_OCM_VKEYTOITEM(func) \
2094 if (uMsg == OCM_VKEYTOITEM) \
2096 SetMsgHandled(TRUE); \
2097 lResult = (LRESULT)func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), (HWND)lParam); \
2098 if(IsMsgHandled()) \
2103#define MSG_OCM_CHARTOITEM(func) \
2104 if (uMsg == OCM_CHARTOITEM) \
2106 SetMsgHandled(TRUE); \
2107 lResult = (LRESULT)func((UINT)LOWORD(wParam), (UINT)HIWORD(wParam), (HWND)lParam); \
2108 if(IsMsgHandled()) \
2113#define MSG_OCM_HSCROLL(func) \
2114 if (uMsg == OCM_HSCROLL) \
2116 SetMsgHandled(TRUE); \
2117 func((int)LOWORD(wParam), (short)HIWORD(wParam), (HWND)lParam); \
2119 if(IsMsgHandled()) \
2124#define MSG_OCM_VSCROLL(func) \
2125 if (uMsg == OCM_VSCROLL) \
2127 SetMsgHandled(TRUE); \
2128 func((int)LOWORD(wParam), (short)HIWORD(wParam), (HWND)lParam); \
2130 if(IsMsgHandled()) \
2135#define MSG_OCM_CTLCOLOREDIT(func) \
2136 if (uMsg == OCM_CTLCOLOREDIT) \
2138 SetMsgHandled(TRUE); \
2139 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
2140 if(IsMsgHandled()) \
2145#define MSG_OCM_CTLCOLORLISTBOX(func) \
2146 if (uMsg == OCM_CTLCOLORLISTBOX) \
2148 SetMsgHandled(TRUE); \
2149 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
2150 if(IsMsgHandled()) \
2155#define MSG_OCM_CTLCOLORBTN(func) \
2156 if (uMsg == OCM_CTLCOLORBTN) \
2158 SetMsgHandled(TRUE); \
2159 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
2160 if(IsMsgHandled()) \
2165#define MSG_OCM_CTLCOLORDLG(func) \
2166 if (uMsg == OCM_CTLCOLORDLG) \
2168 SetMsgHandled(TRUE); \
2169 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
2170 if(IsMsgHandled()) \
2175#define MSG_OCM_CTLCOLORSCROLLBAR(func) \
2176 if (uMsg == OCM_CTLCOLORSCROLLBAR) \
2178 SetMsgHandled(TRUE); \
2179 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
2180 if(IsMsgHandled()) \
2185#define MSG_OCM_CTLCOLORSTATIC(func) \
2186 if (uMsg == OCM_CTLCOLORSTATIC) \
2188 SetMsgHandled(TRUE); \
2189 lResult = (LRESULT)func((HDC)wParam, (HWND)lParam); \
2190 if(IsMsgHandled()) \
2198#define MSG_WM_CLEAR(func) \
2199 if (uMsg == WM_CLEAR) \
2201 SetMsgHandled(TRUE); \
2204 if(IsMsgHandled()) \
2209#define MSG_WM_COPY(func) \
2210 if (uMsg == WM_COPY) \
2212 SetMsgHandled(TRUE); \
2215 if(IsMsgHandled()) \
2220#define MSG_WM_CUT(func) \
2221 if (uMsg == WM_CUT) \
2223 SetMsgHandled(TRUE); \
2226 if(IsMsgHandled()) \
2231#define MSG_WM_PASTE(func) \
2232 if (uMsg == WM_PASTE) \
2234 SetMsgHandled(TRUE); \
2237 if(IsMsgHandled()) \
2242#define MSG_WM_UNDO(func) \
2243 if (uMsg == WM_UNDO) \
2245 SetMsgHandled(TRUE); \
2248 if(IsMsgHandled()) \
2256#define MESSAGE_HANDLER_EX(msg, func) \
2259 SetMsgHandled(TRUE); \
2260 lResult = func(uMsg, wParam, lParam); \
2261 if(IsMsgHandled()) \
2266#define MESSAGE_RANGE_HANDLER_EX(msgFirst, msgLast, func) \
2267 if(uMsg >= msgFirst && uMsg <= msgLast) \
2269 SetMsgHandled(TRUE); \
2270 lResult = func(uMsg, wParam, lParam); \
2271 if(IsMsgHandled()) \
2279#define COMMAND_HANDLER_EX(id, code, func) \
2280 if (uMsg == WM_COMMAND && code == HIWORD(wParam) && id == LOWORD(wParam)) \
2282 SetMsgHandled(TRUE); \
2283 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2285 if(IsMsgHandled()) \
2290#define COMMAND_ID_HANDLER_EX(id, func) \
2291 if (uMsg == WM_COMMAND && id == LOWORD(wParam)) \
2293 SetMsgHandled(TRUE); \
2294 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2296 if(IsMsgHandled()) \
2301#define COMMAND_CODE_HANDLER_EX(code, func) \
2302 if (uMsg == WM_COMMAND && code == HIWORD(wParam)) \
2304 SetMsgHandled(TRUE); \
2305 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2307 if(IsMsgHandled()) \
2312#define NOTIFY_HANDLER_EX(id, cd, func) \
2313 if (uMsg == WM_NOTIFY && cd == ((LPNMHDR)lParam)->code && id == ((LPNMHDR)lParam)->idFrom) \
2315 SetMsgHandled(TRUE); \
2316 lResult = func((LPNMHDR)lParam); \
2317 if(IsMsgHandled()) \
2322#define NOTIFY_ID_HANDLER_EX(id, func) \
2323 if (uMsg == WM_NOTIFY && id == ((LPNMHDR)lParam)->idFrom) \
2325 SetMsgHandled(TRUE); \
2326 lResult = func((LPNMHDR)lParam); \
2327 if(IsMsgHandled()) \
2332#define NOTIFY_CODE_HANDLER_EX(cd, func) \
2333 if (uMsg == WM_NOTIFY && cd == ((LPNMHDR)lParam)->code) \
2335 SetMsgHandled(TRUE); \
2336 lResult = func((LPNMHDR)lParam); \
2337 if(IsMsgHandled()) \
2342#define COMMAND_RANGE_HANDLER_EX(idFirst, idLast, func) \
2343 if(uMsg == WM_COMMAND && LOWORD(wParam) >= idFirst && LOWORD(wParam) <= idLast) \
2345 SetMsgHandled(TRUE); \
2346 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2348 if(IsMsgHandled()) \
2353#define COMMAND_RANGE_CODE_HANDLER_EX(idFirst, idLast, code, func) \
2354 if(uMsg == WM_COMMAND && code == HIWORD(wParam) && LOWORD(wParam) >= idFirst && LOWORD(wParam) <= idLast) \
2356 SetMsgHandled(TRUE); \
2357 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2359 if(IsMsgHandled()) \
2364#define NOTIFY_RANGE_HANDLER_EX(idFirst, idLast, func) \
2365 if(uMsg == WM_NOTIFY && ((LPNMHDR)lParam)->idFrom >= idFirst && ((LPNMHDR)lParam)->idFrom <= idLast) \
2367 SetMsgHandled(TRUE); \
2368 lResult = func((LPNMHDR)lParam); \
2369 if(IsMsgHandled()) \
2374#define NOTIFY_RANGE_CODE_HANDLER_EX(idFirst, idLast, cd, func) \
2375 if(uMsg == WM_NOTIFY && cd == ((LPNMHDR)lParam)->code && ((LPNMHDR)lParam)->idFrom >= idFirst && ((LPNMHDR)lParam)->idFrom <= idLast) \
2377 SetMsgHandled(TRUE); \
2378 lResult = func((LPNMHDR)lParam); \
2379 if(IsMsgHandled()) \
2384#define REFLECTED_COMMAND_HANDLER_EX(id, code, func) \
2385 if (uMsg == OCM_COMMAND && code == HIWORD(wParam) && id == LOWORD(wParam)) \
2387 SetMsgHandled(TRUE); \
2388 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2390 if(IsMsgHandled()) \
2395#define REFLECTED_COMMAND_ID_HANDLER_EX(id, func) \
2396 if (uMsg == OCM_COMMAND && id == LOWORD(wParam)) \
2398 SetMsgHandled(TRUE); \
2399 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2401 if(IsMsgHandled()) \
2406#define REFLECTED_COMMAND_CODE_HANDLER_EX(code, func) \
2407 if (uMsg == OCM_COMMAND && code == HIWORD(wParam)) \
2409 SetMsgHandled(TRUE); \
2410 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2412 if(IsMsgHandled()) \
2417#define REFLECTED_NOTIFY_HANDLER_EX(id, cd, func) \
2418 if (uMsg == OCM_NOTIFY && cd == ((LPNMHDR)lParam)->code && id == ((LPNMHDR)lParam)->idFrom) \
2420 SetMsgHandled(TRUE); \
2421 lResult = func((LPNMHDR)lParam); \
2422 if(IsMsgHandled()) \
2427#define REFLECTED_NOTIFY_ID_HANDLER_EX(id, func) \
2428 if (uMsg == OCM_NOTIFY && id == ((LPNMHDR)lParam)->idFrom) \
2430 SetMsgHandled(TRUE); \
2431 lResult = func((LPNMHDR)lParam); \
2432 if(IsMsgHandled()) \
2437#define REFLECTED_NOTIFY_CODE_HANDLER_EX(cd, func) \
2438 if (uMsg == OCM_NOTIFY && cd == ((LPNMHDR)lParam)->code) \
2440 SetMsgHandled(TRUE); \
2441 lResult = func((LPNMHDR)lParam); \
2442 if(IsMsgHandled()) \
2447#define REFLECTED_COMMAND_RANGE_HANDLER_EX(idFirst, idLast, func) \
2448 if(uMsg == OCM_COMMAND && LOWORD(wParam) >= idFirst && LOWORD(wParam) <= idLast) \
2450 SetMsgHandled(TRUE); \
2451 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2453 if(IsMsgHandled()) \
2458#define REFLECTED_COMMAND_RANGE_CODE_HANDLER_EX(idFirst, idLast, code, func) \
2459 if(uMsg == OCM_COMMAND && code == HIWORD(wParam) && LOWORD(wParam) >= idFirst && LOWORD(wParam) <= idLast) \
2461 SetMsgHandled(TRUE); \
2462 func((UINT)HIWORD(wParam), (int)LOWORD(wParam), (HWND)lParam); \
2464 if(IsMsgHandled()) \
2469#define REFLECTED_NOTIFY_RANGE_HANDLER_EX(idFirst, idLast, func) \
2470 if(uMsg == OCM_NOTIFY && ((LPNMHDR)lParam)->idFrom >= idFirst && ((LPNMHDR)lParam)->idFrom <= idLast) \
2472 SetMsgHandled(TRUE); \
2473 lResult = func((LPNMHDR)lParam); \
2474 if(IsMsgHandled()) \
2479#define REFLECTED_NOTIFY_RANGE_CODE_HANDLER_EX(idFirst, idLast, cd, func) \
2480 if(uMsg == OCM_NOTIFY && cd == ((LPNMHDR)lParam)->code && ((LPNMHDR)lParam)->idFrom >= idFirst && ((LPNMHDR)lParam)->idFrom <= idLast) \
2482 SetMsgHandled(TRUE); \
2483 lResult = func((LPNMHDR)lParam); \
2484 if(IsMsgHandled()) \